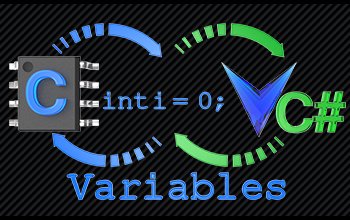
Basic Variable Binding Example
This example shows how a variable can be created and bind to target model.
Description
This example shows how a variable can be created in the Target and configured to be bound to a corresponding variable on the Target Model and then used by the host.
Click here to download complete Project File Click here to download getting started Project File
char SIM_LEDState; // Variable to store the value of LED state
// This will bind to Virtual Device LED control variable
void LEDOn(char _LEDState) // Function to Set the LED State
{
SIM_LEDState = _LEDState;
}
char SIM_SevenSegmentValue; // Variable to store the value of Seven Segment
// This will bind to Virtual Device Seven Segment control variable
char UpdatedSevenSegment(char UserValue) // Function to Update Seven Segment as per User value
{
SIM_SevenSegmentValue = UserValue + 48;
return SIM_SevenSegmentValue;
}
unsigned int SIM_Knob; // Variable to store the value of Knob or Potentiometer
// This will bind to Virtual Device Knob and Gauge
unsigned int ReadKnob(void) // Function to read Knob or Potentiometer Value
{
return SIM_Knob;
}
char i = 0;
void TimerISR(void) // Hardware Timer ISR
{
SIM_LEDState = ~SIM_LEDState; // This will be call at every 1 Sec
// Toggle the LED state at every 1 Sec
UpdatedSevenSegment(i++); // This will update the SSD with incremented value of i(0->9) at every 1 Sec
if (i == 9) // When i becomes equal to 9, Reset i = 0
i = 0; // Because in one SSD we can only display one number at a Time
}
void InitVirtuoso()
{
#ifdef _WINDOWS
InitializeVirtuoso();
#endif //_WINDOWS
}
void main()
{
InitVirtuoso();
LEDOn(1); // Function to turn on the LED
while (1)
{
}
}